I created a new page/space for my side projects (still WIP), where I will try to add all my side projects I worked or still work on.
It will mainly describe shortly the project itself and why I started that project. The code will be hosted at Github, so that everyone can potentially participate on that project.
GoAcccess Nginx Analysis
Few days ago, I installed GoAcccess which is an (open source real-time web log analyzer and interactive viewer that runs in a terminal in *nix systems or through your browser.), to analyze my NGINX access logs.
Main reason for that was to identify which files drain the traffic by asking me, do I need to move the libs to CDN to improve the performance.
But I was surprised by something else: Bots/Crawlers.
I mean all of us know, that the internet will be constantly scanned by crawlers like Google or Bing, and by malicious bots.
But I didn’t expect, that my blog was scanned around 300 times a day by malicious bots.
Here are just few examples from my access logs:
Insomnia GraphQL File Upload Scalar
Uploading a File with Upload Scalar and Insomnia
Uploading a File with “Upload” Scalar isn’t a big problem if you are using library like graphql-upload. But if you like just to try/simulate it with Insomnia, you need to know what happens under to hood.
In fact, you will send a multipart form request to the GraphQL server.
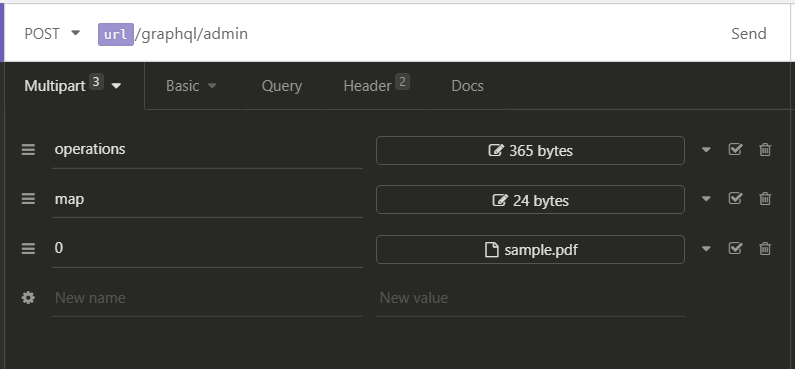
It is split in three parts (be careful, naming is important):
- operations, which contains the GraphQL query and variables
1 | { |
- map, which maps the file you would like to upload 0, with the GraphQL variable name file
- You can extend it, if you like to upload multiple files
1 | {"0":["variables.file"]} |
- 0, refers to the file, which you selected and would like to upload
Note: operations and map has the type “Text (Multi-line)” and not “File”.
Python Logging Benchmark
If you have a heavy logging application, you might want to take into consideration to use the %-format strings for logging.
The Python logging module recommends to use the %-format.
But I guess most of the developers will prefer the f-string format, which came with the Python 3.6 release, because it simply easier to read.
My question was: Which impact does it have on performance? In case, we use logging with string concatenation.
So, I wrote a short script to “benchmark” it (and yes, I know it is not a proper benchmark).
Compared was: logging and concatenation with ‘+’ vs formatting with % vs f-strings, over multiple runs in a different order of the benchmark functions. So that, we can at least exclude the cold start issue.
Benchmark Diagram
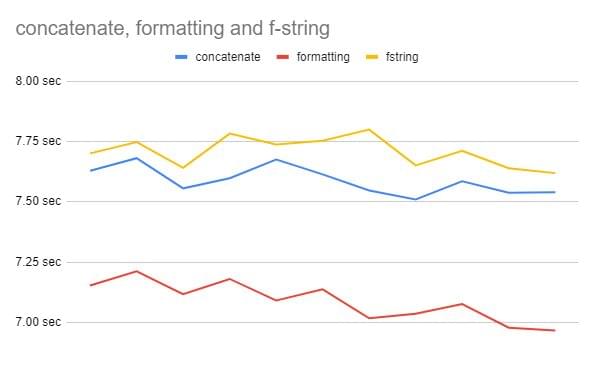
If we take an average of all measuring points and compare them with each other, we would come to the result:
formatting with % | concatenation with + | f-strings |
---|---|---|
7.087545455 sec | 7.588454545 sec | 7.707727273 sec |
100% | 107.06% | 108.75% |
That means that, formatting with % is 8.75% faster than with f-string or 7.06% faster than with concatenation. At least in the combination with “deactivated” logging.
The reason for that is probably, because the string will be concatenated anyway, even if we don’t log anything. But in case of formatting with %, the string will be not concatenated.
Python Common Style Guidelines
One of the best practices is, to define a common style guideline. So that it would be much easier to understand a codebase when all the code in it, is in a consistent style. Specially if you are working in a team. PEP (Python Enhancement Proposals) provides a good starting point to define those style guide.
Here are some highlights which are mostly from PEP 8, but also from other best practices:
Naming Conventions
Avoid one letter variables like x and y (Except in very short code blocks). Give your variables and functions a meaningful name and use follow naming convention:
Variables, functions, packages and modules names should be:
1
lower_case_with_underscores
Classes and Exceptions
1
CamelCase
Protected methods
1
_single_leading_underscore
Private methods
1
__double_leading_underscore
Constants
1
ALL_CAPS_WITH_UNDERSCORES